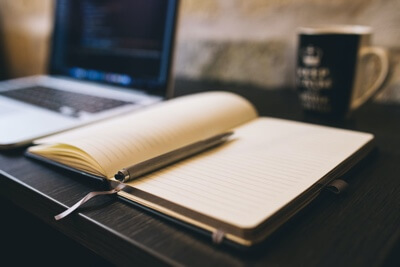
Back when Dinosaurs ruled the earth, developers had these things called API references. They were physical books (made of paper) which contained thousands upon thousands of pages of nothing but documentation for APIs. If you wanted to do something, and you didn’t know how, you either asked the wise old developer that was working with you or you looked up the answer in the API reference. Thankfully I never had to experience that, I mean seriously, how old do you think I am? But what I do remember is the bad old days when search engines weren’t very programming language friendly and you couldn’t even search for anything with special symbols in it because all of the search engines just stripped it all out! Searching for code was quite hard!
Once Google came along things changed. Not only was Google very friendly to programming languages, but it actually returned relevant results to our queries. Over time, the amount of online programming info flourished, and all was good. Well, all was good if you were to leverage the tools that you have at your disposal. And let me be clear, Google was the single most powerful development tool ever created, and probably still is (insert Bing joke here).
So I was plowing through reams of code today (I wish) and I came across a place where someone was manually parsing a connection string in order to extract its contents. I chuckled a bit (you gotta stay light hearted about some of this stuff) and then I went to Google and typed in "C# Parse Connection String". Hmmm, that first answer looked pretty darn good. The first result was the MSDN API reference for SqlConnectionStringBuilder… which turned out to be exactly what I was looking for. Pretty sweet. And to think, a class exists to parse a connection string! Who would have thought? Well, I for one, and I hope that you would think that too. This is what I like to call "a well known problem". It is a problem that a bajillion other people are almost certainly to have come across in their programming adventures, and so someone else has most likely solved it, and probably posted the answer online! Just in case you found this while searching for connection string parsing in C#, I’ll post the answer here:
var sqlConnectionStringBuilder = new SqlConnectionStringBuilder(); sqlConnectionStringBuilder.ConnectionString = "Data Source=myServerAddress;Initial Catalog=myDataBase;User Id=myUsername;Password=myPassword;"; Console.WriteLine(sqlConnectionStringBuilder.InitialCatalog); Console.WriteLine(sqlConnectionStringBuilder.DataSource); Console.WriteLine(sqlConnectionStringBuilder.UserID);
The funny thing about this is that just last night I was chatting (literally, in a chat program) with my buddy Dave Ward and this exact topic came up. How come people don’t do a simple Google search whenever they come to a well known problem? His comment was "How many times has Path.Combine been rewritten?" He has an excellent point, it has probably been rewritten about a million times. And it is a complete waste of time. Although Path.Combine does have a few funky behaviors that could probably be improved upon Don’t believe me? Try doing this:
Path.Combine(@"C:\Path\", @"\OtherPath");
But anyways… whenever you come across a problem like say combining paths, parsing a connection string (or a query string!), or a csv file, make sure you do a few quick searches before you decide to write code to do your own. Sure it may seem easy to parse a connection string, but what happens when you are looking for "Default Catalog" in the connection string and someone throws in a connection string that uses "Database" instead? I bet the framework parser will account for that, but unless you spent your time and due diligence, yours probably does not.
So I implore you, get out there and do a search before you give up and write code…You’d be amazed at what you’ll find lurking in the .NET framework, and the world of code that exists out there in the open source community. I’ll leave you with a few quick examples of what you might find just in the .net framework:
- Parse connection string: System.Data.SqlClient.SqlConnectionStringBuilder
- Parse query string: System.Web.HttpUtility.ParseQueryString
- Parse CSV file: Microsoft.VisualBasic.FileIO.TextFieldParser
- Generate Temporary File Name: System.IO.Path.GetTempFileName
- Time Code: System.Diagnostics.Stopwatch: stop getting the current time and trying to subtract it. This has a much higher precision!
- Coerce Types: System.ComponentModel.TypeConverter
- Check if a string is null or empty: String.IsNullOrEmpty
- Parse Any Uri: System.Uri
- Basic Data Structures: System.Collections.Generic.LinkedList, Queue, Stack: I’ve seen people try to emulate these with lists!
- Add list of items to another list: System.Collections.Generic.List.AddRange: Don’t loop through another list adding items!
- Watch for changes in a file system: System.IO.FileSystemWatcher
There are probably a ton more examples of these, but I’ll let you explore a bit more and let me know which ones you find! So get out there, use Google, Yahoo, Bing, Ask, etc… and find some code that you can reuse!
Loved the article? Hated it? Didn’t even read it?
We’d love to hear from you.
Good day!
I’ve used some of these before, and they are really helpful, especially Path.Combine(). But I did encounter a problem with this, combining a network path, in which an IP address was specified. The method threw an exception. It was a long time ago, but I ended up concatenating the path explicitly. Is this really by design?
Yeah,
To my surprise i tried to feed google with the keyword "C# Parse Connection String", it only returned one entry which is where I am writing comment now. 😉
I really enjoy reading your posts.
What is this "paper" you speak of?
You know, I *still* reinvent these wheels sometimes too.
Just a few months ago, I realized that my PostBackRitalin control was manually building its JSON settings string with string concatenation. Meanwhile, the control has a dependency on ASP.NET AJAX, which means that the framework’s JavaScriptSerializer is available.
/facepalm.
I deleted probably three dozen LoC and replaced with one. On the one hand, that refactor felt great, but it was also depressing that I had given myself the opportunity in the first place.
@configurator Don’t worry about it, it has been deprecated.
@Dave We all do, but that is an excellent example!
You know, I saw this headline in my RSS feed and thought "Oh, geeze!"
I clicked through, thinking it was going to be some rave review for a new application. Boy, was I wrong! And I completely agree with you, Google searches are the backbone of my development career!
@Misty Well, I am glad that my link-bait powers are strong 🙂
Great list. I just wanted to add an alternate to "Watch for changes in a file system" for medium trust ASP.NET environments. (i.e. GoDaddy) You can use the Cache object to watch for file changes when you can’t use System.IO.FileSystemWatcher.
I have 3 words for you: legacy, legacy, legacy.
I needed to parse a CSV. I would’ve loved to use
Microsoft.VisualBasic.FileIO.TextFieldParser
but alas, "This class is new in the .NET Framework version 2.0" and my company’s codebase is still used with some .NET 1.1 programs.
So, I’m often forced to re-invent the wheel wether I like it or not.
@Brad But if you don’t have the tool available to you, then you aren’t really doing anything wrong. This post is all about leveraging tools that are available to us. A class that isn’t in your version of the framework really isn’t available to you. But there is always reflector! 🙂
I could not agree with you more. Google is your friend! I actually landed my current job because of Google.
In my interview I was asked the question, "How would you rate your skills in C#, 1-5?"
I replied "4", even though I had never seen a line of C# code.
He asked me why I rated myself a 4 and I told him, "Programming is a skill, once you know how, everything else is just syntax and I can just Google the rest."
@rauhr Awesome, nice Colbert impersonation there. Chutzpah!
What about Bing? Developers always have to chase the new shiny. Overall, I give it a MEH out of 5 stars for development related searching.
[quote]just last night I was chatting (literally, in a chat program[/quote]
ah, the internet age.
You should probably check out filehelpers for CSV parsing too (http://filehelpers.sourceforge.net/)
I think one stumbling block can often be that coders don’t know what to search for in Google, i.e. they don’t actually know the technical definition of what they’re doing, or they don’t know how to phrase it.
Umm…I certainly hope this is obvious information to most.
@Really Knowing and doing are two *very* different things. I’ve seen people reinvent the wheel on seemingly obvious problems many times before, so while this may be obvious, it is not always done.
I agree actually…my personal favorite is coming across a homegrown implementation of UrlEncode().
Honestly to me this is a classic example of "common sense isn’t so common". That fact that someone may actually try write/do something that has likely been solved countless times without looking for it – to me is just troubling. Granted, I’m in the "search for everything before you do it" camp.
Don’t forget http://www.searchdotnet.com/ , which should give you more focused results.
Great post Justin. I am still mostly maintaining .NET 1.1 projects at work with Visual Studio 2003 and after this post just looking thru the namespaces you listed I see that I am constantly reinventing the wheel on several of the projects instead of getting new features added to the legacy code base and its definitively time for a change. I’m going to try and move these projects immediately to .NET 2.0 at the very least. It really sucks using outdated tech.
Hi
Thanks for the great post. I have been trying to get people and developers alike to understand this simple process of searching on google. By far it is the most powerful development tool available.
What I love the most about it is, copying and pasting in weird error messages that you pick up along your programming adventures. and wiola! I always find a fix within the first page of google results.
Good post. Hopefully more people will learn to see the value in taking this route.
Great post – Just subscriped to your RSS feed.. Thanks
Great post – Just subscriped to your RSS feed.. Thanks