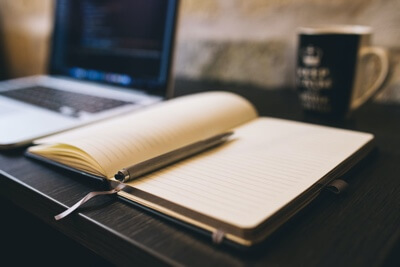
In the last part of this series we talked a little bit about Scala and why I think it is an interesting language. We also spent a good amount of time getting the boring work out of the way of setting up an environment for us to work in. We are not going to start off with the IDE though. The reason for this is that it is much easier for us to start to get our bearings within Scala by using Scala’s REPL (Read Evaluate Print Loop).
If you have come from C# or Java then it is quite possible that you have never even used a REPL before, and in fact, it is fairly uncommon for a static compiled language to have a REPL. If you have any experience with dynamic languages though, then you are probably intimately familiar with the concept. The idea is that you will enter in statements and they will be dynamically evaluated and the results printed to the screen. This may sound strange and useless at first, until you realize that you can enter multiple commands and they are all loaded up into the same environment. You can load files and libraries and do all of the things you would do in a normal development environment, but in a more interactive fashion.
If you followed the last post in this series then you will already have the Scala REPL in your path, in which case all you need to do is fire up a command line and enter “scala” (you should see something similar to below):
If you have not yet put this in your path, then you’ll just need to go to wherever you have unzipped the Scala binaries and go into the “bin” folder. For example, on my system, scala.bat is located in “C:\tools\scala-2.7.6.final\bin\”.
Before we get started with Scala’s syntax, let me point you to the Scala Language Spec so that you can bookmark it in case you have questions in the future. It is not the best reference you can use (there are several good books), but it will most likely provide you with answers to any questions you might have.
Once you are at the above screen, the first thing I want you to type in is:
val x: Int = 1;
Before we get any further, let me first say that line is very bad Scala, and I’ll tell you why in a bit. But first, let’s break down this line just a little bit.
Variables as Values
The first part that we have is “val” which tells scala that we are declaring a value. A value type is immutable, which means that we cannot change it. So later on, if we try to do this:
x = 2;
We get an error from Scala telling us that we cannot reassign to a value. The concept of built in immutability in Scala is very important. As we will learn later on, this is a core part of the languages design.
Type Declaration
The second thing that you see in this line is “x: Int”. This is telling Scala that the variable “x” is of type “Int”. Notice that the “I” in “Int” is capitalized. Following the conventions of many OO languages, this would signal that this is a reference type, and in this case that is exactly correct. Everything in Scala is an object.
Perhaps the most interesting thing though about this part of the line is that we can leave off the “: Int” altogether. Just like like a line of C# that would look like this:
var x = 1;
The line in Scala could easily be rewritten to look like this:
val x = 1;
Ahhh, much better! Scala has very good type inference which allows it to figure out what type “x” is so that you don’t have to restate it.
Assignment
The next part of this simple statement is the assignment. This works exactly as it would in C# only, as we said earlier, because we used “val” this variable cannot be reassigned. Scala also forces all local variables like this to be initialized, so even if we specified a type, we could not enter something like this:
val x: Int;
It is bad form in most languages to leave variables uninitialized, but in Scala it is enforced by the compiler.
Semicolons
The last thing you will notice is the semicolon at the end of the line. While you may be used to it with C#, in Scala it is actually not needed, and most often it is not used. If we were to follow good practice, then the line that we wrote originally would actually look like this:
val x = 1
Nice and succinct. With this simple line Scala now knows that we want an immutable variable “x” with the type of Int and a value of 1.
Simple Operators
So now if we wanted to, we could declare a second variable called “y” and add “x” and “y” together.
val x = 1 val y = 2 val z = x + y
Great, that looks almost exactly like the corresponding C#:
var x = 1; var y = 2; var z = x + y;
But something very different is going on here. In the C# code we are using the assignment operator to assign the numbers to each variable, and then we are using the addition operator to add them together. In Scala it looks as if we are doing the same thing, but when it comes to the addition operator, we are doing something slightly different.
You see, in Scala since everything is an object the need for special operators in the language is diminished. Think about if you had an integer type which was an object, you could declare a “+” method, and then do this:
val z = x.+(y)
So you see, we are just calling the “+” operator on the “x” object and passing “y” into it. And this is actually what is happening in Scala! Instead of having operators for things like this, Scala provides special rules for certain types of method calls. If a method call has only a single parameter then you are able to leave the “.” and the parentheses off the method call which leaves you with this:
val z = x + y
So even though it looks like we are using the “+” operator, we are actually just calling a method on the Int object. So if we declare a string type and use the “+” operator, then we are doing the exact same thing:
val greeting = "hello " + "justin" val greeting = "hello ".+("justin")
This has some big implications within the language, because it allows you to build additions to the language that look like first class citizens, but are in fact just part of the language’s libraries.
Wrap-up
In the entry we have seen how you would use Scala’s REPL to declare a simple immutable type and then perform some basic operations. You have learned that in Scala, everything is an object, and then combined this with Scala’s method syntax to allow for methods to replace operators in the language. In the next entry we are going to take a look at how you would create mutable variables, and then look at how you would define some simple control structures in Scala. I hope you enjoyed, and I hope you’ll come back for the next entry!
Loved the article? Hated it? Didn’t even read it?
We’d love to hear from you.
Justin,
Its good to see .NET folks exploring Scala. As you detail, the type inference and immutable vals are great features.
If your looking at getting deep into Scala, I would recommend looking at partial functions and for comprehensions – they rock out and will change the way you think about programming when (or hopefully if) you return to .NET.
Cheers,
Timothy
lift and akka committer
@Timothy Thanks, I’m enjoying going through Scala quite a bit, it is a very interesting language.
And yes, I am certainly going to check out partial functions and comprehensions, but just don’t assume that because I am .NET guy that I don’t know what partial application is. 🙂 http://www.codethinked.com/post/2007/12/26/Lambdas-and-Closures-and-Currying-Oh-my!-%28Part-8%29.aspx
And as far as comprehensions go, Linq provides most of the same functionality in .NET. It leverages delayed execution (also using the ‘yield" keyword http://www.codethinked.com/post/2008/06/Delayed-execution-and-quot3byield-returnquot3b.aspx )
So yes, I am very intrigued to be looking into Scala, but I think that a lot of people that are outside of the .NET world see it as a toy and not a serious programming environment. I would encourage you to peek a little bit on my side of the fence. 🙂
I never assumed you didnt know what a lambda was 😉 I know LINQ has them… I code C# from time to time as well… just dont assume because im a OSS junkie I dont know anything about .NET (touché!!)
Did you know that the Scala compiler was actually designed to have multiple outputs and that the CLR backend for the compiler is on the move again? The original design of the compiler was such that it could output bytecode for a variety of VMs!
Scala is awesome; firstly because of the language, and secondly because of the community: everyone is very smart and its just such a great place to be.
Enjoy 🙂
@Timothy Excellent point! I’m a bit defensive, I am a .NET developer after all 🙂
And yes, I certainly hope that the CLR version of Scala catches up and gets some support on the .NET platform. I’m not holding my breath, but it would be great if we got some Scala love.
I look forward to my adventures in Scala and hopefully you’ll follow my series and let me know if I make any stupid mistakes. Thanks!
Hey, no worries 🙂
One thing I would say however, don’t use Scala 2.7.6… it was a broken release by EPFL and is known to be bad (we refused to use it for lift)
I recommend using the new (fixed) release of 2.7.7… 2.8 is just around the corner so if you want to be on the edge, just use that as it should be released in a few months time and has a totally new collections library and numerous different improvements and stabilisations.
Good luck!
@Timothy Awesome, I’ll grab 2.8. I haven’t gotten too far into anything which will break if I switch versions. Thanks!