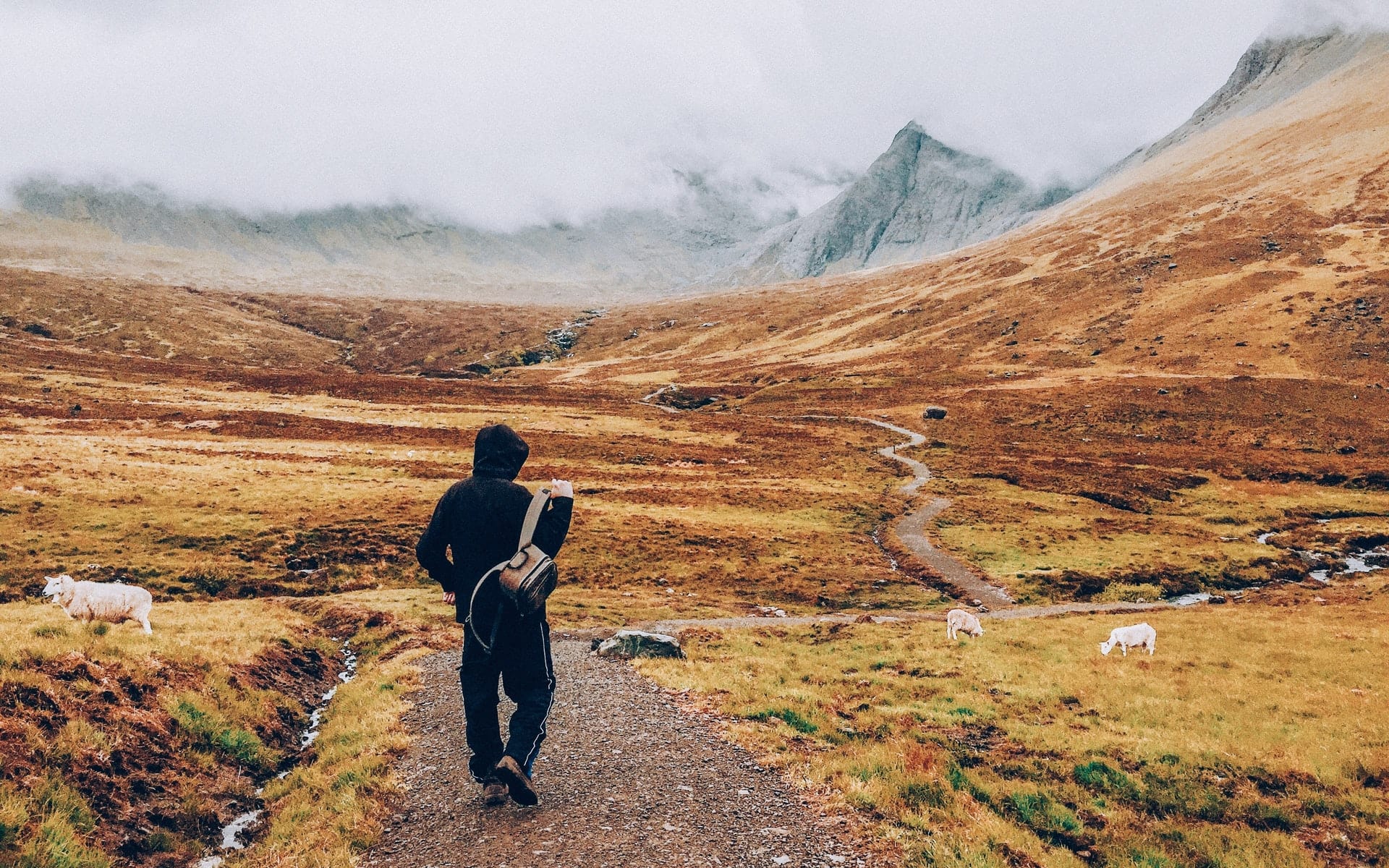
You’ve landed a new gig, and today’s your first day on the job. Congratulations! You’ve gotten your work-issued laptop, set your desktop wallpaper, installed your development tools, signed into GitHub, pulled down a copy of the repository you’ll be working on, and made sure you can run the app locally.
Uh. Now what?
Specifically, how do you get to know this new code base? Which parts of it are brilliant, and which parts of it are going to give you nightmares? There are ways to explore new code that will help you hit the ground running once you’re assigned your first Trello card.
Here are a few tricks that I use. Note that my workflows are pretty Ruby- and Rails-centric, as that’s what I work with most often; however, there are analogous techniques in other languages and frameworks for just about everything I’ll write about below.
Find the big files
I try to follow Sandi Metz’s rules for developers when I’m writing code. One that I particularly like is keeping classes to 100 lines or less. Anything longer is usually an indication that the class can be split up into smaller classes.
When I start a new project, I like to find the biggest classes and read through them. They usually hide some of the gnarliest code (i.e., technical debt) in the repo. Here’s a quick command to print a list of files with their line counts:
$ find . -path './db' -prune -o -path './config' -prune -o -name '*.rb' | xargs wc -l
I have this set to an alias named linecount in my ZSH config.
Run this command, scroll back through the output, and look for any really big files. Anything over 100 lines is worth looking at, but anything over 500 should be a red flag and is really worth investigating.
Read the tests
This is especially helpful if your project has extensive integration tests. You can use the trick above to scan the tests to find the largest test files. Often, though not always, those large test files correspond with the primary functionality of the application. Knowing what the previous developers felt deserved the most testing gives you a good idea of what makes this software valuable and where you’ll likely be spending most of your time.
Check the dependencies
Open up the Gemfile or package.json file. Read through both, and Google any packages that don’t ring a bell. You can learn a lot about what the app does by the packages that are being pulled in, including how users are authenticated (and whether multi-factor auth is supported), what third-party services are being used, and what UI frameworks are supported.
Look at the non-application code
With Rails, this means digging through the lib directory. Developers have a tendency to stuff code in here when they can’t find another place to put it.
Also, run rake -T
to see a list of rake tasks that have been added to the project. Is there a lot of stale code in these tasks? Are there any tasks that appear to be cron jobs? If so, what’re they doing?
Finally, look for any other directories that have been added to the project that aren’t part of the standard application structure. Those directories could be hiding interesting or curious behavior. For Rails, I look for directories like app/services, app/decorators, and app/serializers.
Look through the application’s routes
Run rake routes
and scan the output. Are there any /api routes? Are those API routes versioned? How long is the entire list? The longer the route list, the more complex the application.
Run static analysis tools
For Ruby, that means Rubocop and Brakeman. I don’t do this on every project, but these tools can give you a feel for how closely previous developers adhered to standards, both in terms of code formatting and security.
Scan the git history
This one’s pretty obvious but worth mentioning. It’s the entire app’s history in reverse chronological order! Even if the commit messages aren’t very detailed, you can still get a pretty good idea of what’s been going on over the last few months.
Ask questions
Finally, when you’re done with your research, you’ll probably have questions. Here are a few things I usually ask:
- If you’re on a project that’s been around for a while and something doesn’t behave the way you think it should, ask why. There’s often a story behind it that will provide context for not just the code but the team and, if there is one, the client. Example: “I noticed the migrations don’t run. Why do we have to reset our database using the schema file instead of just running the migrations?”
- Unless you’ve already figured it out by browsing the code, ask how the app is deployed. This might not be pertinent now (or ever if there’s a dedicated devops team), but it will tell you more about the hosting environment, which can affect how you code solutions to certain problems.
- “What should I test?” This isn’t a way to weasel out of writing tests. Some teams have different philosophies. Some favor integration tests over controller and view tests. Some want tests across the whole stack.
The steps above should fill the rest of your first day (if not your first couple of days). By the end of it, though, you should have enough of an understanding of the application to start confidently making changes without the fear of messing anything up.
Do you have other techniques you use to get familiar with new code? Drop them in the comments below – I’d love to hear them.
Loved the article? Hated it? Didn’t even read it?
We’d love to hear from you.