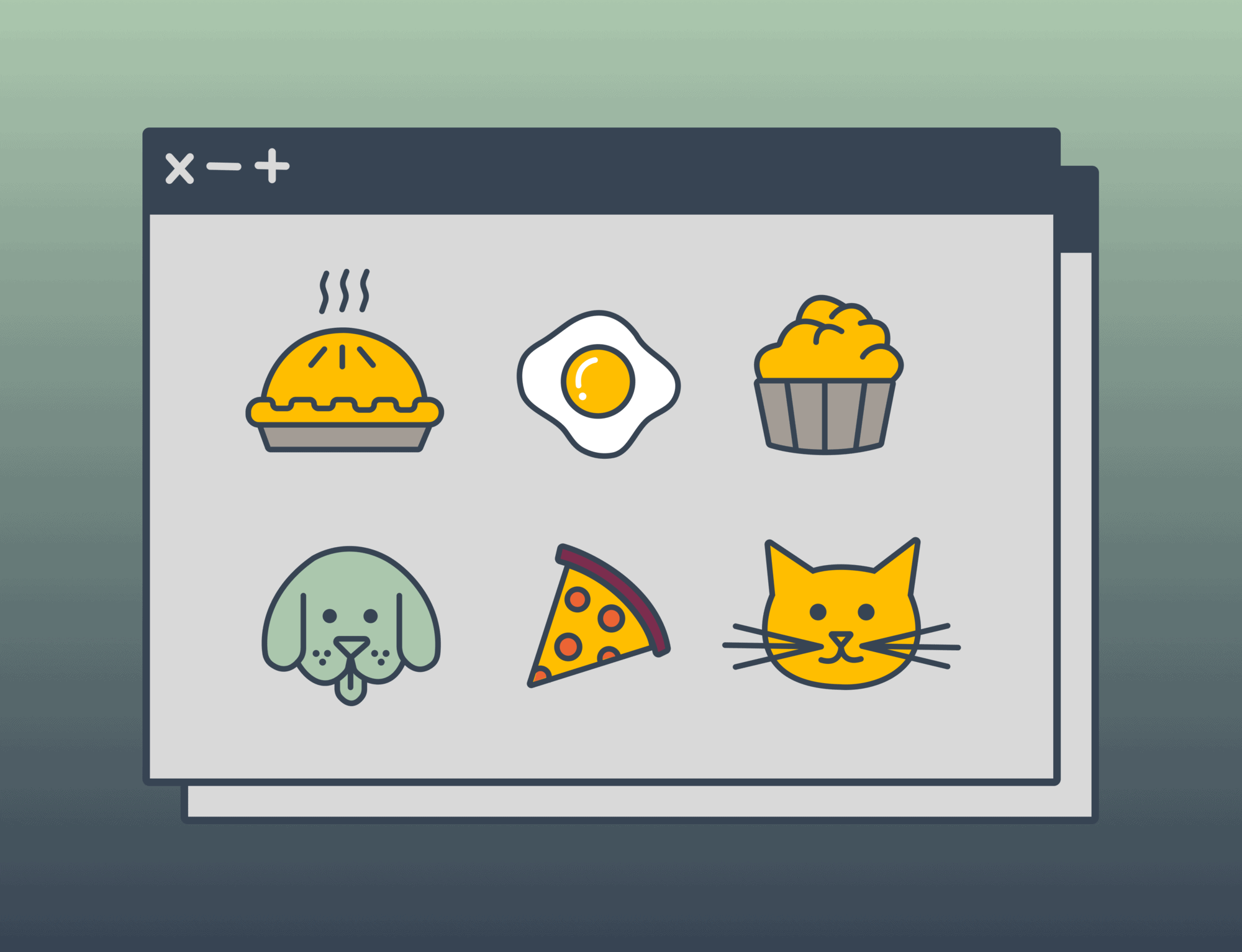
FooBar is FooBad
FooBar is a metasyntactic variable. A “specific word or set of words identified as a placeholder in computer science”, per wikipedia. It’s the most abstract stand-in imaginable, the formless platonic ideal of a Programming Thing. It can morph into a variable, method or class with the barest change of capitalization and spacing. Like “widget”, it’s a catch-all generic term that lets you ignore the specifics and focus on the process.
And it’s overused.
Concrete > Abstract
Human brains were built to deal with real things. We can deal with unreal things, but it takes a little bit of brainpower. And when learning a new language or tool, brainpower is in scarce supply. Too often, `FooBar` is used in tutorials when almost anything else would be better.
Say I’d like to teach Python inheritance to a new learner.
# Inheritance
class Foo:
def baz(self):
print("FooBaz!")
class Bar(Foo):
def baz(self):
print("BarBaz!")
A novice learner will have no idea what the above code is doing. Is it `Bar` inheriting from `Foo` or vice versa? If it seems obvious to you that’s because you already understand the code! It makes sense because we already know how it works. Classic curse of knowledge. Why force learners to keep track of where Foo comes before Bar instead of focusing on the actual lesson?
Compare that to this example using concrete, real-world, non-abstract placeholders:
# Inheritance
class Animal:
def speak(self):
print("")
class Dog(Animal):
def speak(self):
print("Bark!")
This is trite and reductive. But it works. It’s immediately clear which way the inheritance runs. Your brain leverages its considerable real-world knowledge to provide context instead of mentally juggling meaningless placeholder words. As a bonus, you effortlessly see that the Dog
class is a noun/thing and the speak()
method is verb/action.
Concrete Is Better for Memory
Even if a learner parses your tutorial, will they remember it? The brain remembers concrete words better than abstract ones. Imagine a cherry pie, hot steaming, with a scoop of ice cream melting down the side. Can you see it?
Now try to imagine a “Foo”… Can you see it?
Yeah, me neither.
Concrete examples are also more unique. Animal::Dog
is more salient than Foo::Bar
in the same way “John is a baker” is easier to remember than someone’s name is “John Baker”. It’s called the Baker-Baker Effect. Your brain is full of empty interchangeable labels like Foo
, Bar
, John Smith
. But something with relationships, with dynamics and semantic meaning? That stands out.
Concrete Is Extensible
Lets add more examples to our tutorial.
Sticking to Foo, I suppose I could dig into the Metasyntactic variable wikipedia page and use foobar, foo, bar, baz, qux, quux, corge, grault, garply, waldo, fred, plugh, xyzzy
and thud
.
# Inheritance
class Foo:
def qux(self):
print("FooQux!")
class Bar(Foo):
def qux(self):
print("BarQux!")
class Baz(Foo):
def qux(self):
print("BazQux!")
But by then, we’ve strayed from ‘beginner demo’ to ‘occult lore’. And the code is harder to understand than before!
Using a concrete example on the other hand…
# Inheritance
class Animal:
def speak(self):
print("")
class Dog(Animal):
def speak(self):
print("Bark!")
class Cat(Animal):
def speak(self):
print("Meow!")
Extension is easy and the lesson is reinforced rather than muddied.
Exercise for the reader: See if you can rewrite these python examples on multiple inheritance in a non-foobar’d way.
Better Than Foo
Fortunately, there are alternatives out there. The classic intro Animal
, or Vehicle
and their attending subclasses. Or might I suggest using Python’s convention of spam
, eggs
, and hams
? A five-year old could intuit what eggs = 3
means.
There’s also cryptography’s Alice and Bob and co. Not only are they people (concrete), but there’s an ordinal mapping in the alphabetization of their names. As an added bonus, the name/role alliteration aids in recall. (Mallory is a malicious attacker. Trudy is an intruder)
New Proposal: Pies
Personally, I think Pies
make excellent example variables. They’re concrete, have categories (Sweet, Savory), subtypes (Fruit, Berry, Meat, Cream) and edge cases (Pizza Pies, Mud Pies).
# Pies
fruit = ['cherry', 'apple', 'fig', 'jam']
meat = ['pork', 'ham', 'chicken', 'shepherd']
nut = ['pecan', 'walnut']
pizza = ['cheese', 'pepperoni', 'hawaiian']
other = ['mud']
They also come baked-in with a variety of easy-to-grasp methods and attributes like slice()
, bake()
, bake_time
or price
. All of which can be implicitly understood.
Though if pies aren’t your thing, there’s a whole world of concrete things to choose from.
Maybe breads?
['bun', 'roll', 'bagel', 'scone', 'muffin', 'pita', 'naan']
Conclusion
I’m not holding my breath for FooBar to be abolished. It has its use cases. It’s short, easy, abstract, and (most importantly) established.
Mentally mapping concrete concepts is hard. Analogies are tricky and full of false assumptions. Maps are not the territory. You’re trying to collapse life in all its complexity to something recognizable but not overly reductive or inaccurate. But the solution is not to confuse abstractness for clarity.
For tutorials, extended docs and beginner audiences, skip FooBar. Use concrete concepts instead, preferably something distinct that can be mapped onto the problem space. And if it gives implicit hierarchy, relationships, or noun/verb hinting, so much the better.
Use FooBar when you’re trying to focus on the pure abstract case without extra assumptions cluttering the syntax. Use it in your console, debuggers, and when you’re talking to experienced programmers.
But for anything longer than a brief snippet, avoid it.
Loved the article? Hated it? Didn’t even read it?
We’d love to hear from you.
Not to mention that it brings to mind the meaning of the military-derived homonym fubar. Fubar certainly isn’t the type of code you want to write!
THANK YOU! When I was learning programming in college (at the turn of the millennium) instructors were always using “foo” for variable names and I didn’t know why. (Frequently without the corresponding “bar”.) I thought it was some old pop culture reference from before I was born, like when they would use the names Bob & Carol & Ted & Alice as names in database samples.
And why always “foo” along with “bar” instead of “fu”? Even knowledge of the military acronym (“Fouled” Up Beyond All Recognition) doesn’t help in understanding code samples using these variable names.
Years ago the official Python documentation was nearly as bad, littered with obscure Monty Python references that were meaningless to anyone that didn’t get the references. They’ve cut back on those a lot and only retained ones like you mentioned above that don’t require you to get the reference to understand the code sample.
I hope people start taking your advice.
Foobar was a Microsoft joke in the original QW_Basic programmers guide. That is FUBAR is a military term for F**ked Up Beyond All Recognition.
GW-BASIC is a dialect of the BASIC programming language developed by Microsoft from IBM BASICA. circa 1983.
Longest running joke in IT
Foobar was used long before MS existed, see The New Hackers Dictionary
I always used lasagna and cake as my examples. I would bring a box of frozen lasagna and a big batch of cupcakes to class. Some cupcakes were strawberry, others chocolate. My first question was how are they the same – other than they are edible. By the end, showing a bake() class which had an ingredients (list), temperature, container, fill container, etc. properties, I more or less showed they were the same except for how the properties were set. And that a basic cupcake recipe could be easily subclassed to chocolate or strawberry just be adding the additional ingredient (and maybe reducing the sugar a bit.) It made it easier to understand that a recipe can’t be eaten, but once it’s instantiated – the instantiated (cupcake) could be. This let me explain the differences between a class and object (and how to figure out what to change), encapsulation, inheritance, and polymorphism all in one easy to digest session….. At the end, they all got to eat the cupcakes…..